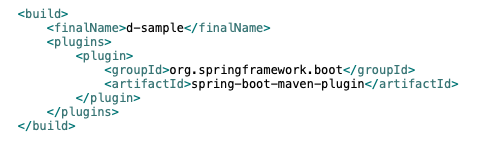
[SpringBoot] war 배포
·
IT/Spring
2018년 초가을 대전에서 모 프로젝트를 진행했을 때, 고객님께서 무조건 톰캣에 war로 배포를 해달라는 요청이 있었습니다. 프로젝트 막판까지 개발은 SpringBoot로 개발하고 Jar 배포로 다 합의된 사항이었지만.. 마지막에 고객님의 강력한 요구로 war로 묶어서 톰캣에 올렸던 추억을 생각하면서 어떤 부분을 고쳐야 war로 묶을 수 있는지 보겠습니다. pom.xml 에서 jar 부분을 war 로 변경을 해줍니다. war로 패키징 한 후에 톰캣에 올라갈 수 있게 spring-boot-starter-tomcat 을 추가해줍니다. org.springframework.boot spring-boot-starter-tomcat provided 그러고 나서 Build 부분에 finalName 추가해 줍니다. d..